문제 1093
import java.io.*;
public class Main {
//첫 번째 줄에 출석 번호를 부른 횟수인 정수 n이 입력된다. (1 ~ 10000)
//두 번째 줄에는 무작위로 부른 n개의 번호(1 ~ 23)가 입력된다.
//1번부터 번호가 불린 횟수를 순서대로 공백으로 구분하여 한 줄로 출력한다.
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int n = Integer.parseInt(br.readLine());
String[] a = br.readLine().split(" ");
int[] b = new int[23];
for (int i = 0; i<n; i++){
b[Integer.parseInt(a[i])-1]++;
}
for (int i = 0; i<23;i++){
bw.write(String.format("%s ", b[i]));
}
bw.flush();
}
}
문제 1094
import java.io.*;
public class Main {
// 번호를 부른 횟수(n, 1 ~ 10000)가 첫 줄에 입력된다.
// n개의 랜덤 번호(k, 1 ~ 23)가 두 번째 줄에 공백을 사이에 두고 순서대로 입력된다.
// 출석을 부른 번호 순서를 바꾸어 공백을 두고 출력한다.
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int n = Integer.parseInt(br.readLine());
String[] a = br.readLine().split(" ");
for (int i = a.length-1; i>=0; i--){
bw.write(String.format("%s ", a[i]));
}
bw.flush();
}
}
문제 1095
import java.io.*;
public class Main {
//번호를 부른 횟수(n, 1 ~ 10000)가 첫 줄에 입력된다.
//n개의 랜덤 번호(k, 1 ~ 23)가 두 번째 줄에 공백을 사이에 두고 순서대로 입력된다.
//출석을 부른 번호 중에 가장 빠른 번호를 1개만 출력한다.
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int min = 24;
int n = Integer.parseInt(br.readLine());
String[] a = br.readLine().split(" ");
for (int i = 0; i<a.length; i++){
if (Integer.parseInt(a[i])<min) min = Integer.parseInt(a[i]);
}
bw.write(String.valueOf(min));
bw.flush();
}
}
문제 1096
import java.io.*;
public class Main {
/*
바둑판에 올려 놓을 흰 돌의 개수(n)가 첫 줄에 입력된다.
둘째 줄 부터 n+1 번째 줄까지 힌 돌을 놓을 좌표(x, y)가 n줄 입력된다.
n은 10이하의 자연수이고 x, y 좌표는 1 ~ 19 까지이며, 같은 좌표는 입력되지 않는다.
*/
/*
흰 돌이 올려진 바둑판의 상황을 출력한다.
흰 돌이 있는 위치는 1, 없는 곳은 0으로 출력한다.
*/
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int n = Integer.parseInt(br.readLine());
int[][] a = new int[19][19]; // 0으로 초기화된 2차원 배열 생성
for (int i=0;i<n;i++){
String[] s = br.readLine().split(" ");
a[Integer.parseInt(s[0])-1][Integer.parseInt(s[1])-1] = 1;
}
for (int x=0; x<19; x++){
for (int y=0; y<19; y++) {
bw.write(String.format(a[x][y]+" "));
}
bw.write("\n");
}
bw.flush();
}
}
문제 1097
import java.io.*;
public class Main {
/*
바둑알이 깔려 있는 상황이 19 * 19 크기의 정수값으로 입력된다. v
십자 뒤집기 횟수(n)가 입력된다.v
십자 뒤집기 좌표가 횟수(n) 만큼 입력된다. 단, n은 10이하의 자연수이다.v
*/
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int[][] a = new int[19][19];
for (int i = 0; i<19; i++){
String[] s = br.readLine().split(" ");
for (int j = 0; j<19; j++){
a[i][j] = Integer.parseInt(s[j]);
}
}
int n = Integer.parseInt(br.readLine());
for (int i = 0; i<n; i++){
String[] ss = br.readLine().split(" ");
int x = Integer.parseInt(ss[0]) -1;
int y = Integer.parseInt(ss[1]) -1;
for(int j=0; j<19; j++) //가로 줄 바꾸기
{
if(a[x][j]==1) a[x][j]= 0;
else a[x][j]= 1;
}
for(int j=0; j<19; j++) //세로 줄 바꾸기
{
if(a[j][y]==1) a[j][y]=0;
else a[j][y] = 1;
}
}
for (int x=0; x<19; x++){
for (int y=0; y<19; y++) {
bw.write(String.format(a[x][y]+" "));
}
bw.write("\n");
}
bw.flush();
}
}
문제 1098
import java.io.*;
public class Main {
/*
첫 줄에 격자판의 세로(h), 가로(w) 가 공백을 두고 입력되고,
두 번째 줄에 놓을 수 있는 막대의 개수(n)
세 번째 줄부터 각 막대의 길이(l), 방향(d), 좌표(x, y)가 입력된다. 단, d = 0 or 1
*/
//막대에 의해 가려진 경우 1, 아닌 경우 0으로 출력한다.
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
String[] s = br.readLine().split(" ");
int h = Integer.parseInt(s[0]);
int w = Integer.parseInt(s[1]);
// int n = Integer.parseInt(br.readLine()); //NumberFormatException
String[] S = br.readLine().split(" ");
int n = Integer.parseInt(S[0]); // 입력의 첫 번째 값만 정수로 변환
// 2차원 배열 생성
int[][] a = new int[h][w];
for (int i = 0; i<n; i++){
String[] ss = br.readLine().split(" ");
int l = Integer.parseInt(ss[0]);
int d = Integer.parseInt(ss[1]);
int x = Integer.parseInt(ss[2])-1;
int y = Integer.parseInt(ss[3])-1;
if (x >= 0 && x < h && y >= 0 && y < w) { // 유효한 범위 내에서 배열에 값을 설정
// 막대 넓이 d가 0일때 == 가로로 놓일때(x고정)
if (d==0){
for (int j = y; j<y+l; j++){
a[x][j] = 1;
}
}
// 막대 넓이 d가 1일때 == 세로로 놓일때(y고정)
else {
for (int j = x; j<x+l; j++){
a[j][y] = 1;
}
}
}
}
for (int i = 0; i < h; i++) {
for (int j = 0; j < w; j++) {
bw.write(a[i][j] + " ");
}
bw.write("\n");
}
bw.flush();
}
}
문제 1099
import java.io.*;
import java.util.*;
public class Main {
// 10*10 크기의 미로 상자의 구조와 먹이의 위치가 입력된다. v
// 성실한 개미가 이동한 경로를 9로 표시해 출력한다.
// 0(갈 수 있는 곳), 1(벽 또는 장애물), 먹이가 2
// 개미는 (2, 2)에서 출발
// 종료 조건 :
// 맨 아래의 가장 오른쪽에 도착한 경우, 더 이상 움직일 수 없는 경우, 먹이를 찾은 경우
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int[][] a = new int[10][10];
for (int i = 0; i<10; i++){
StringTokenizer st= new StringTokenizer(br.readLine());
for (int j = 0;j<10;j++){
a[i][j] = Integer.parseInt(st.nextToken());
}
}
int x = 1;
int y = 1;
while(true){
//먹이를 찾은 경우
if (a[x][y]==2){
a[x][y]=9;
break;
}
a[x][y] = 9; //이동한 경로 표시
// 개미는 오른쪽 또는 아래쪽으로만 움직임
// [오른쪽 이동] 갈 수 있는 곳이면 다음 반복문에 그곳으로 이동
if(a[x][y+1]==0 || a[x][y+1]==2){
y++;
}
// [아래로 이동]
else if(a[x][y+1]==1){
if (a[x+1][y]==0||a[x+1][y]==2){
x++;
}
// 오른쪽, 아래로도 이동 안되는 경우
else if (a[x+1][y] == 1)
break;
}
}
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
bw.write(a[i][j] + " ");
}
bw.write("\n");
}
bw.flush();
}
}
더보기
드디어 코드업 기초 100제가 끝났다!
2차원 배열 문제가 갑자기 어려워져서 좀 당황했지만, 오랜만에 머리도 굴리고 재미있었다!!
다음 글로 코드업 후기를 작성해보겠어요~~ 아싸 드디어!!
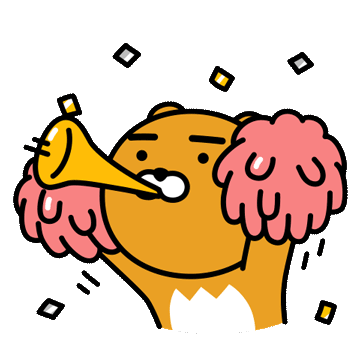
'코딩 테스트 일지 📒' 카테고리의 다른 글
[백준] 2438 별 찍기 - 1 | 구현 | 브론즈 Ⅴ | JAVA 💡StringBuilder 사용 (0) | 2024.09.16 |
---|---|
[코드업/JAVA] 기초 100제 완료 후기! 🎉 (0) | 2024.08.26 |
[코드업/JAVA] 기초 100제 7. 종합 1078-1092 🐘 (0) | 2024.08.24 |
[코드업/JAVA] 기초 100제 6. 조건/선택실행구조&반복실행구조 1065-1077 🐘 (0) | 2024.08.23 |
[코드업/JAVA] 기초 100제 5. 논리연산&비트단위논리연산 1053-1064 🐘 (0) | 2024.08.22 |